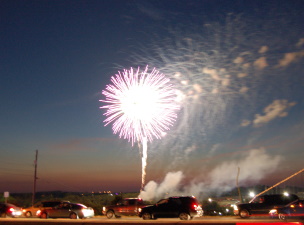
September 22, 2018
Automatically posting messages to slack
S
QL Server supports SQL Mail for email notifications, and traditionally I’ve used SQL Mail to keep myself apprised of various server issues.
Slack is a great alternative to email, and a lot of folks make extensive use of slack. I thought it would be nice to receive notifications from SQL Server in a slack channel, and so started looking into what would be necessary to programmatically generate messages to slack.
Assuming you have created a slack application and have an authorization token, and assuming that you wish to post the message to a channel (posting to specific users is a subject for another day), here is a routine that you can use that uses the slack postMessage API to send a message to a specified channel.
public string PostMessageRaw(
string token,
string channel,
string message)
{
string responseText = string.Empty;
var request = (HttpWebRequest)WebRequest.Create("https://slack.com/api/chat.postMessage");
string encodedMessage =message.Replace("\\", "\\\\"); //slack requires that slashes be escaped.
StringBuilder postData = new StringBuilder();
postData.Append("{")
.AppendFormat("\"channel\":\"{0}\",", channel)
.AppendFormat("\"text\":\"{0}\"", encodedMessage)
.Append("}");
var data = Encoding.UTF8.GetBytes(postData.ToString());
request.Method = "POST";
request.ContentType = "application/json; charset=utf-8";
request.Headers.Add(string.Format("Authorization: Bearer {0}", token));
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
responseText = new StreamReader(response.GetResponseStream()).ReadToEnd();
return responseText;
}