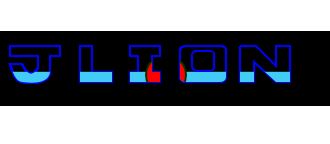
Clipping is a little like photoshop paths. You use the HTML5 api to draw shapes and then you can use clipping to show content only inside the boundaries of that path.
In a nutshell, here’s how it works.
First you’ll need a canvas element, perhaps something like this:
<canvas id="clipcanv" width="700" height="75">Canvas is not supported</canvas>
Get the context of the canvas element:
var ctx = canvas.getContext('2d');
Save the state onto the stack so that the clipping region doesn’t apply to subsequent updates of the canvas.
ctx.save();
Draw a shape (here, the outside of the letter “O”)
ctx.lineTo(x, y + 31);
ctx.quadraticCurveTo(x + 0 , y + 35 , x + 5 , y + 36);
ctx.lineTo(x + 39, y + 36);
ctx.quadraticCurveTo(x + 46, y + 36, x + 46, y + 31);
ctx.lineTo(x + 46, y + 5);
ctx.quadraticCurveTo(x + 47, y + 1, x + 41, y);
ctx.lineTo(x + 5, y);
ctx.quadraticCurveTo(x + 0, y + 1, x, y + 5);
ctx.lineWidth = _lineWidth;
ctx.strokeStyle = 'blue';
ctx.stroke();
ctx.closePath();
Use the Clip method to indicate that this path will be used for clipping.
ctx.clip();
Only the portion of anything that you draw on the canvas that is visible within the clipping region will now be visible. For example, a circle:
ctx.save();
ctx.beginPath();
ctx.arc(x, y, 20, 0, 2 * Math.PI);
ctx.fillStyle = 'red';
ctx.fill();
ctx.lineWidth = 0;
ctx.strokeStyle = '#003300';
ctx.stroke();
ctx.closePath();
ctx.restore();
And finally another call to Restore is needed to indicate that we’re done with clipping.
ctx.restore();